Introduction
Deep Q-Networks (DQN) have emerged as a groundbreaking technique in the realm of reinforcement learning, paving the way for machines to learn and make decisions in complex environments. Combining the power of deep neural networks with the principles of Q-learning, DQN has proven to be a robust solution for training agents to perform tasks ranging from playing classic video games to controlling autonomous vehicles. In this article, we will delve into the intricacies of Deep Q-Networks, exploring their architecture, training process, and real-world applications.
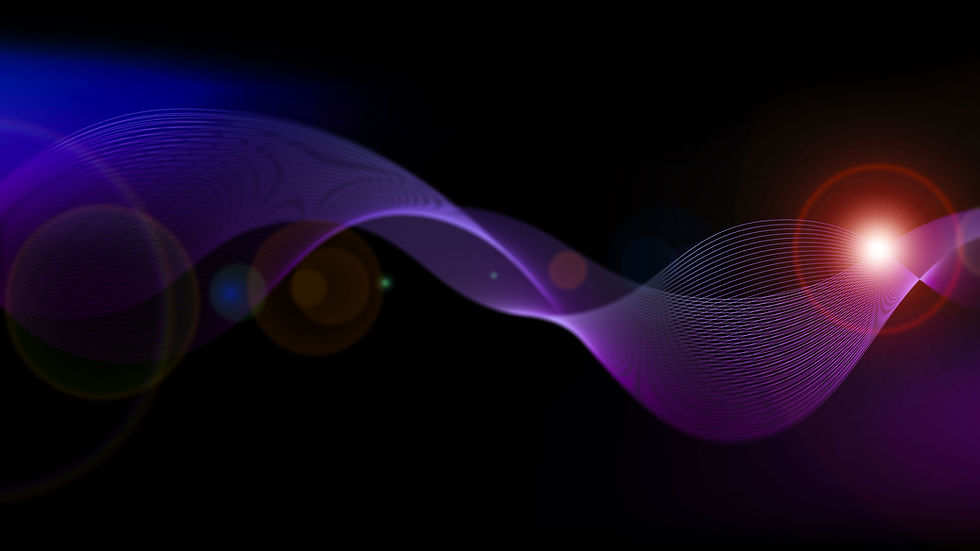
Understanding Deep Q-Networks
Deep Q-Networks are an extension of Q-learning, a model-free reinforcement learning algorithm. Q-learning aims to learn a policy, a strategy that guides an agent's actions in an environment to maximize cumulative rewards over time. The Q-value represents the expected cumulative reward of taking a particular action in a specific state and following a certain policy.
DQN takes this concept to the next level by employing deep neural networks to approximate the Q-function. The neural network is trained to predict Q-values for each possible action in a given state. The model is updated iteratively using a combination of temporal difference learning and experience replay.
Key Components of DQN
1. Experience Replay:
DQN utilizes experience replay to break the correlation between consecutive samples. It stores past experiences (state, action, reward, next state) in a replay buffer and samples randomly during the training process. This helps stabilize and improve the learning process.
2. Target Q-Network:
To improve the stability of the training process, DQN employs a target Q-network, which is a separate copy of the Q-network with fixed parameters. This target network is periodically updated to approximate the current Q-values.
3. Loss Function:
The loss function used for training DQN is derived from the temporal difference error, measuring the difference between the predicted Q-values and the target Q-values. The model is then optimized to minimize this loss.
Example Implementation:
Let's implement a simple DQN using Python and TensorFlow. For brevity, we'll use a basic example of training an agent to play the classic game CartPole from the OpenAI Gym environment.
# Import necessary libraries
import tensorflow as tf
import numpy as np
import gym
# Create the Q-network
model = tf.keras.Sequential([
tf.keras.layers.Dense(24, input_shape=(4,), activation='relu'),
tf.keras.layers.Dense(24, activation='relu'),
tf.keras.layers.Dense(2, activation='linear') # 2 actions in CartPole: left or right
])
# Compile the model
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=0.001),
loss='mse') # Mean Squared Error loss for Q-learning
# Define other parameters
epsilon = 1.0 # Exploration-exploitation trade-off
epsilon_decay = 0.995
min_epsilon = 0.01
gamma = 0.99 # Discount factor for future rewards
# Initialize the environment
env = gym.make('CartPole-v1')
# Training loop
for episode in range(1000):
state = env.reset()
state = np.reshape(state, [1, 4]) # Reshape state for the model input
total_reward = 0
while True:
# Epsilon-greedy strategy for action selection
if np.random.rand() < epsilon:
action = env.action_space.sample() # Explore
else:
q_values = model.predict(state)
action = np.argmax(q_values[0]) # Exploit
# Take the chosen action and observe the next state and reward
next_state, reward, done, _ = env.step(action)
next_state = np.reshape(next_state, [1, 4])
# Update Q-value using the Bellman equation
target = reward + gamma * np.max(model.predict(next_state)[0])
q_values = model.predict(state)
q_values[0][action] = target
# Train the model on the updated Q-values
model.fit(state, q_values, epochs=1, verbose=0)
total_reward += reward
state = next_state
if done:
break
# Decay exploration rate
epsilon = max(min_epsilon, epsilon * epsilon_decay)
# Print the total reward for the episode
print(f"Episode {episode + 1}, Total Reward: {total_reward}")
# Close the environment
env.close()